3D Cube Software Rendering
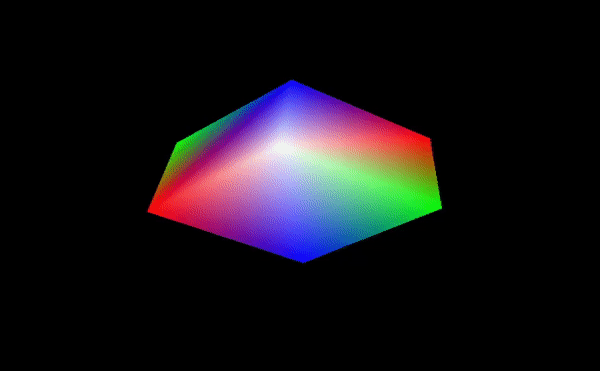
Create a 3D Cube from Scratch
When I say from scrach what I mean is that we are not calling any functions specifically to perform rendering functions from libraries such as Opengl or Direct3D.
Instead on the Windows operating system we have a bitmap which we can change the color values each frame and that is it!
To learn more about 3D graphics I wanted to rasterize a single Cube. Before I could do that I needed to render a triangle.
Render a Triangle
- Perspective Project 3D points to 2D viewing Plane
- Normalize coordiantes between [0,1]
- resize the coordiates to raster space by multipling by the width and height of the window.
- loop over the points that make up the Triangle
- If the point is inside the triangle fill it in with color
- the way to determine whether a point is inside the triangle is found using this equation
- that value returned by each call of the function,also represents the color value. this is known as Barycentric Coordinates
- Multiply the vertex color by the Barycentric coordiate value to determine the color
V3
PerspectiveProject(V3 Point, V3 Camera)
{
V3 result={};
result.X = ((Point.X+Camera.X) * Camera.Z)/(Camera.Z - Point.Z);
result.Y = ((Point.Y+Camera.Y) * Camera.Z)/(Camera.Z - Point.Z);
result.Z = Point.Z;
return result;
}
float
edgeFunction(V2 a,V2 b, V2 c)
{
return(((c.X - a.X)*(b.Y-a.Y))-((c.Y-a.Y)*(b.X-a.X))) ;
}
//p being the point we want to test in raster space
//V0 being a Vertex of the triangle in raster space
//V0 being a Vertex of the triangle in raster space
float w2 =edgeFunction(V0,V1,p);
Rasterize the Cube
Once I was able to rasterize a single trianlge I had to For this project I created wanted to create a 3d Rasterizer
Rotation
Link- When Rotating about an axis you change the other two dimensions, If you rotate on the x axis then the y and z coordiates undergo a translation.
- We are able to calculate a rotated position knowing only the
- Rotation Point, the point we wish to rotate
- Pivot Point, the point which we are rotating about
- Angle of Rotation, the angle for each axis
float deltaZ = rPoint.Z - PivotPoint.Z;
float deltaY = rPoint.Y - PivotPoint.Y;
float magnitude = sqrt((deltaZ*deltaZ)+(deltaY*deltaY));
float existingAngle = atan2(deltaY,deltaZ);
rPoint.Z = PivotPoint.Z + (magnitude*(cosf(AngleOfRotation.X+existingAngle)));
rPoint.Y = PivotPoint.Y + (magnitude*(sinf(AngleOfRotation.X+existingAngle)));